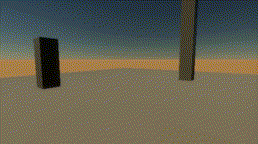
FPSコントローラーの位置と回転をセーブ・ロードしてみます。
using UnityEngine;
using UnityEngine.SceneManagement;
using UnityEngine.UI;
using System.Collections;
public class GameScript2 : MonoBehaviour
{
[SerializeField] Transform player;
[SerializeField] Text text;
string filename;
bool displayingText;
float textAlpha;
// Start is called before the first frame update
void Start()
{
filename = Application.dataPath + "/testsavedata3.xml";
if (System.IO.File.Exists(filename))
{
var data = XmlUtil.Deserialize<SaveData>(filename);
var caracterController = player.GetComponent<CharacterController>();
caracterController.enabled = false; // キャラクターコントローラーをオフ
player.position = data.playerPos; // 位置を適用
player.rotation = data.playerRotation; // 回転を適用
caracterController.enabled = true; // キャラクターコントローラーをオン
}
else
{
Save(); // 保存
}
textAlpha = text.color.a;
text.text = "ロード";
StartCoroutine("DiplayText");
}
private void Update()
{
if (Input.GetKeyDown(KeyCode.E))
{
Save();
StopCoroutine("DiplayText");
text.text = "セーブ";
StartCoroutine("DiplayText");
}
else if (Input.GetKeyDown(KeyCode.R))
{
SceneManager.LoadScene("TestScene2");
}
}
void Save()
{
SaveData data = new SaveData();
data.playerPos = player.position;
data.playerRotation = player.rotation;
XmlUtil.Seialize(filename, data);
}
IEnumerator DiplayText()
{
Color textColor = text.color;
textColor.a = textAlpha;
text.color = textColor;
yield return new WaitForSeconds(.8f);
WaitForSeconds wait = new WaitForSeconds(0.01f);
while (true)
{
yield return wait;
textColor.a -= 0.03f;
text.color = textColor;
if (textColor.a <= 0f)
{
text.text = "";
yield break;
}
}
}
}
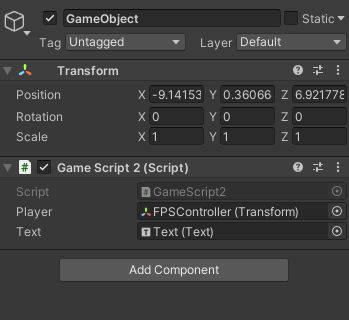
ゲームの進行状況をセーブ/ロードするの方法で、プレイヤーの位置と回転をセーブして、Start()でロードします。セーブデータが無ければ今の状態を保存します。
セーブデータがあるときは、キャラクターコントローラーを無効にしてから、ロードした値をプレイヤーのトランスフォームに代入して、キャラクターコントローラーを有効に戻します。
var caracterController = player.GetComponent<CharacterController>();
caracterController.enabled = false; // キャラクターコントローラーをオフ
player.position = data.playerPos; // 位置を適用
player.rotation = data.playerRotation; // 回転を適用
caracterController.enabled = true; // キャラクターコントローラーをオン
そして、Update()でキー入力によってXMLファイルへの書き込みとシーンのロードを行います。
private void Update()
{
if (Input.GetKeyDown(KeyCode.E))
{
Save();
StopCoroutine("DiplayText");
text.text = "セーブ";
StartCoroutine("DiplayText");
}
else if (Input.GetKeyDown(KeyCode.R))
{
SceneManager.LoadScene("TestScene2");
}
}
void Save()
{
SaveData data = new SaveData();
data.playerPos = player.position;
data.playerRotation = player.rotation;
XmlUtil.Seialize(filename, data);
}
これで、プレイヤーの位置と回転を簡単にセーブ/ロードできました。
テキストは、最初のアルファの値を保存しておいて、コルーチンをスタートしたときに、アルファを元に戻し、その後アルファが0以下になるまで小さい値を繰り返し引き続けることで、ゆっくりと消えるようにしています。
IEnumerator DiplayText()
{
Color textColor = text.color;
textColor.a = textAlpha;
text.color = textColor;
yield return new WaitForSeconds(.8f);
WaitForSeconds wait = new WaitForSeconds(0.01f);
while (true)
{
yield return wait;
textColor.a -= 0.03f;
text.color = textColor;
if (textColor.a <= 0f)
{
text.text = "";
yield break;
}
}
}